03/04/2023 5:32 am
Topic starter
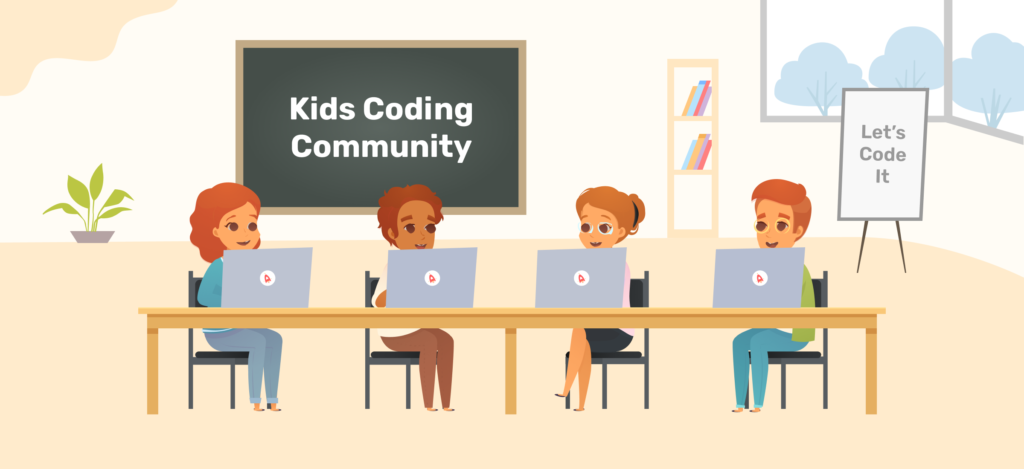
Notifications
Clear all
How do you perform a binary search in a given array?
1
Add a comment
1 Answer
1
03/04/2023 7:53 am

here is a step-by-step guide on how to perform a binary search in a given array:
- Sort the array in ascending or descending order.
- Set two pointers: left pointer (l) at the beginning of the array and right pointer (r) at the end of the array.
- Compute the middle index of the array by taking the average of left and right pointers: mid = (l + r) / 2
- If the target value is equal to the value at the middle index, return the middle index.
- If the target value is less than the value at the middle index, set the right pointer to mid – 1 and go to step 3.
- If the target value is greater than the value at the middle index, set the left pointer to mid + 1 and go to step 3.
- Repeat steps 3 to 6 until the target value is found or the left pointer is greater than the right pointer.
- If the target value is not found, return -1 to indicate that the value is not in the array.
This algorithm has a time complexity of O(log n) because it cuts the search space in half on each iteration, making it very efficient for searching large sorted arrays.
Add a comment
Add a comment
Forum Information
- 22 Forums
- 1,969 Topics
- 5,307 Posts
- 1 Online
- 1,282 Members
Our newest member: TimothySenue
Latest Post: Space bug using " & n b s p ; "
Forum Icons:
Forum contains no unread posts
Forum contains unread posts
Topic Icons:
Not Replied
Replied
Active
Hot
Sticky
Unapproved
Solved
Private
Closed