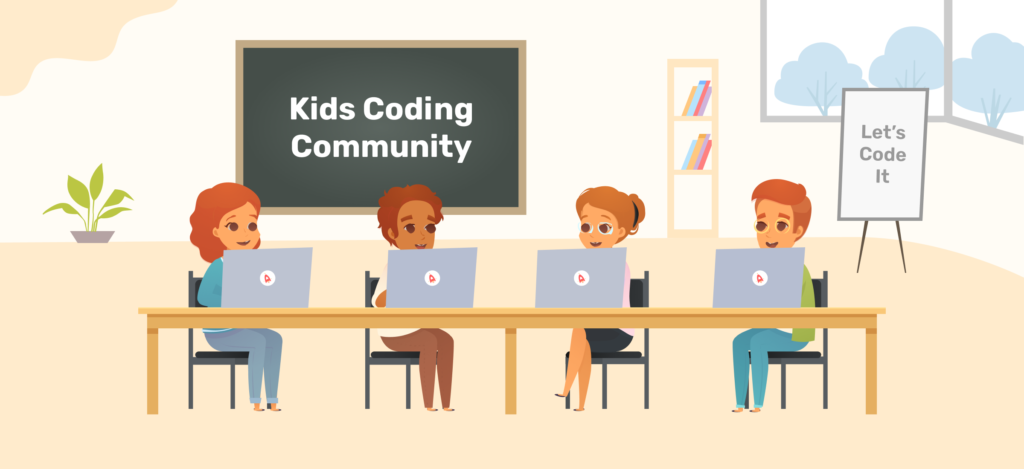
Notifications
Clear all
How to check if a given year is a leap year in Java?
0
06/04/2023 4:48 am
Topic starter
How to check if a given year is a leap year in Java?
Answer
Add a comment
Add a comment
1 Answer
0
06/04/2023 7:37 am

In Java, you can check if a given year is a leap year using the following code:
This code uses the standard rule that a year is a leap year if it is divisible by 4, except for years that are divisible by 100 but not divisible by 400.
To use this method, you can simply call it with the year you want to check as the argument, like this:
public static boolean isLeapYear(int year) { if (year % 4 == 0) { if (year % 100 == 0) { if (year % 400 == 0) { return true; } else { return false; } } else { return true; } } else { return false; } } int year = 2024; if (isLeapYear(year)) { System.out.println(year + " is a leap year"); } else { System.out.println(year + " is not a leap year"); }
Add a comment
Add a comment
Forum Information
- 22 Forums
- 1,969 Topics
- 5,308 Posts
- 0 Online
- 1,283 Members
Our newest member: Thomasnat
Latest Post: Space bug using " & n b s p ; "
Forum Icons:
Forum contains no unread posts
Forum contains unread posts
Topic Icons:
Not Replied
Replied
Active
Hot
Sticky
Unapproved
Solved
Private
Closed